Before you send a PDF document to printer, you may want to preview the printing result in advance. In this article, you will learn how to print preview a PDF document in a Windows Forms application by using PrintPreviewControl and Spire.PDF for .NET.
Create a Windows Forms Application
The Windows Forms application simply consists of a PrintPreviewControl and a button. Once the user clicks the button “Preview”, the PDF document will be loaded and displayed in the PrintPreviewControl.
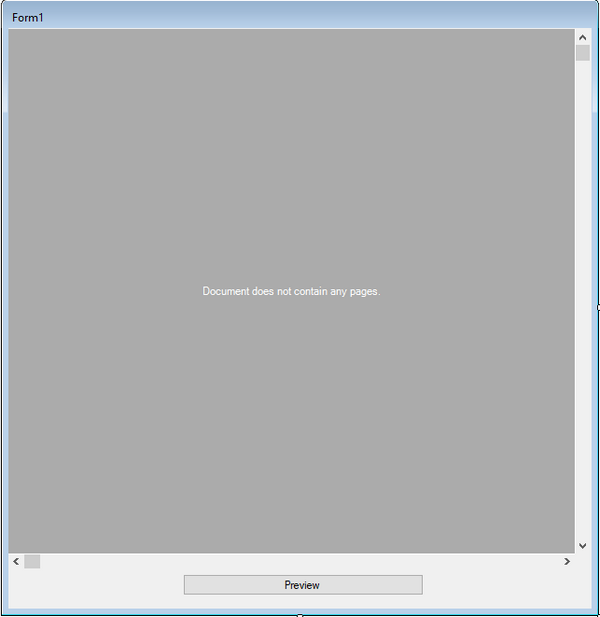
INSTALL SPIRE.PDF
After the Windows Forms application is created, you need to download the latest version of Spire.PDF from this link, and manually add the DLL files in your application as references. Or, you can install it directly via NuGet.

Using the Code
Double click the button “Preview” to add the following code to the click event.
[C#]
using System;
using System.Windows.Forms;
using Spire.Pdf;
namespace PrintPreview
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
//Create a PdfDocument object
PdfDocument document = new PdfDocument();
//Load a sample PDF file
document.LoadFromFile(@"C:\Users\Administrator\Desktop\sample2.pdf");
//Get the page count
int pageCount = document.Pages.Count;
//Set the row number and column number for displaying
this.printPreviewControl1.Rows = (int)Math.Ceiling(pageCount*1.0/3);
this.printPreviewControl1.Columns = 3;
//Call Preview method to print preview PDF in the control
document.Preview(this.printPreviewControl1);
}
}
}
[VB.NET]
Imports System
Imports System.Windows.Forms
Imports Spire.Pdf
Namespace PrintPreview
Public partial Class Form1
Inherits Form
Public Sub New()
InitializeComponent()
End Sub
Private Sub button1_Click(ByVal sender As Object, ByVal e As EventArgs)
'Create a PdfDocument object
Dim document As PdfDocument = New PdfDocument()
'Load a sample PDF file
document.LoadFromFile("C:\Users\Administrator\Desktop\sample2.pdf")
'Get the page count
Dim pageCount As Integer = document.Pages.Count
'Set the row number and column number for displaying
Me.printPreviewControl1.Rows = CType(Math.Ceiling(pageCount*1.0/3), Integer)
Me.printPreviewControl1.Columns = 3
'Call Preview method to print preview PDF in the control
document.Preview(Me.printPreviewControl1)
End Sub
End Class
End Namespace
Run the program, click “Preview” and you’ll get the following output.
